# Media Control
Elementor Core Basic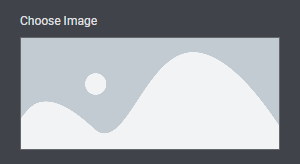
Elementor Media control displays a media chooser section based on the WordPress media library. It allows the user to select an image from the media library.
The control is defined in Control_Media
class which extends Control_Base_Multiple
class.
When using this control, the type
should be set to \Elementor\Controls_Manager::MEDIA
constant.
# Arguments
Name | Type | Default | Description |
---|---|---|---|
type | string | media | The type of the control. |
label | string | The label that appears above of the field. | |
description | string | The description that appears below the field. | |
show_label | bool | true | Whether to display the label. |
label_block | bool | true | Whether to display the label in a separate line. |
separator | string | default | Set the position of the control separator. Available values are default , before and after . default will hide the separator, unless the control type has specific separator settings. before / after will position the separator before/after the control. |
media_types | array | ['image'] | Supported media types. Available values are image , video , svg , application/pdf etc. |
default | array |
Default media values.
|
# Return Value
[
'id' => '',
'url' => '',
]
1
2
3
4
2
3
4
(array
) An array containing image data:
- $id (
int
) Media id. - $url (
string
) Media url.
# Usage
Add an image:
<?php
class Elementor_Test_Widget extends \Elementor\Widget_Base {
protected function register_controls(): void {
$this->start_controls_section(
'content_section',
[
'label' => esc_html__( 'Content', 'textdomain' ),
'tab' => \Elementor\Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'image',
[
'label' => esc_html__( 'Choose Image', 'textdomain' ),
'type' => \Elementor\Controls_Manager::MEDIA,
'default' => [
'url' => \Elementor\Utils::get_placeholder_image_src(),
],
]
);
$this->end_controls_section();
}
protected function render(): void {
$settings = $this->get_settings_for_display();
if ( empty( $settings['image']['url'] ) ) {
return;
}
// Get image URL
echo '<img src="' . $settings['image']['url'] . '">';
// Get image 'thumbnail' by ID
echo wp_get_attachment_image( $settings['image']['id'], 'thumbnail' );
// Get image HTML
$this->add_render_attribute( 'image', 'src', $settings['image']['url'] );
$this->add_render_attribute( 'image', 'alt', \Elementor\Control_Media::get_image_alt( $settings['image'] ) );
$this->add_render_attribute( 'image', 'title', \Elementor\Control_Media::get_image_title( $settings['image'] ) );
$this->add_render_attribute( 'image', 'class', 'my-custom-class' );
echo \Elementor\Group_Control_Image_Size::get_attachment_image_html( $settings, 'thumbnail', 'image' );
}
protected function content_template(): void {
?>
<#
if ( '' === settings.image.url ) {
return;
}
const image = {
id: settings.image.id,
url: settings.image.url,
size: settings.image_size,
dimension: settings.image_custom_dimension,
model: view.getEditModel()
};
const image_url = elementor.imagesManager.getImageUrl( image );
if ( '' === image_url ) {
return;
}
#>
<img src="{{ image_url }}">
<?php
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
Add a video media type to display a self hosted video:
<?php
class Elementor_Test_Widget extends \Elementor\Widget_Base {
protected function register_controls(): void {
$this->start_controls_section(
'content_section',
[
'label' => esc_html__( 'Content', 'textdomain' ),
'tab' => \Elementor\Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'video',
[
'label' => esc_html__( 'Choose Video File', 'textdomain' ),
'type' => \Elementor\Controls_Manager::MEDIA,
'media_types' => [ 'video' ],
'default' => [
'url' => \Elementor\Utils::get_placeholder_image_src(),
],
]
);
$this->end_controls_section();
}
protected function render(): void {
$settings = $this->get_settings_for_display();
$video_url = $settings['video']['url'];
if ( empty( $video_url ) ) {
return;
}
?>
<video src="<?php echo esc_attr( $video_url ); ?>" class="elementor-video"></video>
<?php
}
protected function content_template(): void {
?>
<#
const video_url = settings.video.url;
if ( '' === video_url ) {
return;
}
#>
<video src="{{ video_url }}" class="elementor-video"></video>
<?php
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
Add a PDF file:
<?php
class Elementor_Test_Widget extends \Elementor\Widget_Base {
protected function register_controls(): void {
$this->start_controls_section(
'content_section',
[
'label' => esc_html__( 'Content', 'textdomain' ),
'tab' => \Elementor\Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'pdf',
[
'label' => esc_html__( 'Choose PDF', 'textdomain' ),
'type' => \Elementor\Controls_Manager::MEDIA,
'media_types' => [ 'application/pdf' ],
]
);
$this->end_controls_section();
}
protected function render(): void {
$settings = $this->get_settings_for_display();
$pdf_url = $settings['pdf']['url'];
if ( ! empty( $pdf_url ) ) {
return;
}
?>
<a download href="<?php echo esc_attr( $pdf_url ); ?>">
<?php echo esc_html__( 'Download PDF', 'textdomain' ); ?>
</a>
<?php
}
protected function content_template(): void {
?>
<#
const pdf_url = settings.pdf.url;
if ( '' === pdf_url ) {
return;
}
#>
<a download src="{{ pdf_url }}">
<?php echo esc_html__( 'Download PDF', 'textdomain' ); ?>
</a>
<?php
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56