# Simple Example
Elementor Core IntermediatePutting all these pieces together, we're going to create a simple Elementor widget which will use the native oEmbed functionality (opens new window) of WordPress to auto-embed content from external sites using simple URLs.
# Folder Structure
The addon will have two files. One file for the widget and a main file to register the widget.
elementor-oembed-widget/
|
├─ widgets/
| └─ oembed-widget.php
|
└─ elementor-oembed-widget.php
1
2
3
4
5
6
2
3
4
5
6
# Plugin Files
elementor-oembed-widget.php
<?php
/**
* Plugin Name: Elementor oEmbed Widget
* Description: Auto embed any embbedable content from external URLs into Elementor.
* Plugin URI: https://elementor.com/
* Version: 1.0.0
* Author: Elementor Developer
* Author URI: https://developers.elementor.com/
* Text Domain: elementor-oembed-widget
*
* Requires Plugins: elementor
* Elementor tested up to: 3.21.0
* Elementor Pro tested up to: 3.21.0
*/
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
/**
* Register oEmbed Widget.
*
* Include widget file and register widget class.
*
* @since 1.0.0
* @param \Elementor\Widgets_Manager $widgets_manager Elementor widgets manager.
* @return void
*/
function register_oembed_widget( $widgets_manager ) {
require_once( __DIR__ . '/widgets/oembed-widget.php' );
$widgets_manager->register( new \Elementor_oEmbed_Widget() );
}
add_action( 'elementor/widgets/register', 'register_oembed_widget' );
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
widgets/oembed-widget.php
<?php
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
/**
* Elementor oEmbed Widget.
*
* Elementor widget that inserts an embbedable content into the page, from any given URL.
*
* @since 1.0.0
*/
class Elementor_oEmbed_Widget extends \Elementor\Widget_Base {
/**
* Get widget name.
*
* Retrieve oEmbed widget name.
*
* @since 1.0.0
* @access public
* @return string Widget name.
*/
public function get_name() {
return 'oembed';
}
/**
* Get widget title.
*
* Retrieve oEmbed widget title.
*
* @since 1.0.0
* @access public
* @return string Widget title.
*/
public function get_title() {
return esc_html__( 'oEmbed', 'elementor-oembed-widget' );
}
/**
* Get widget icon.
*
* Retrieve oEmbed widget icon.
*
* @since 1.0.0
* @access public
* @return string Widget icon.
*/
public function get_icon() {
return 'eicon-code';
}
/**
* Get widget categories.
*
* Retrieve the list of categories the oEmbed widget belongs to.
*
* @since 1.0.0
* @access public
* @return array Widget categories.
*/
public function get_categories() {
return [ 'general' ];
}
/**
* Get widget keywords.
*
* Retrieve the list of keywords the oEmbed widget belongs to.
*
* @since 1.0.0
* @access public
* @return array Widget keywords.
*/
public function get_keywords() {
return [ 'oembed', 'url', 'link' ];
}
/**
* Get custom help URL.
*
* Retrieve a URL where the user can get more information about the widget.
*
* @since 1.0.0
* @access public
* @return string Widget help URL.
*/
public function get_custom_help_url() {
return 'https://developers.elementor.com/docs/widgets/';
}
/**
* Register oEmbed widget controls.
*
* Add input fields to allow the user to customize the widget settings.
*
* @since 1.0.0
* @access protected
*/
protected function register_controls() {
$this->start_controls_section(
'content_section',
[
'label' => esc_html__( 'Content', 'elementor-oembed-widget' ),
'tab' => \Elementor\Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'url',
[
'label' => esc_html__( 'URL to embed', 'elementor-oembed-widget' ),
'type' => \Elementor\Controls_Manager::TEXT,
'input_type' => 'url',
'placeholder' => esc_html__( 'https://your-link.com', 'elementor-oembed-widget' ),
]
);
$this->end_controls_section();
}
/**
* Render oEmbed widget output on the frontend.
*
* Written in PHP and used to generate the final HTML.
*
* @since 1.0.0
* @access protected
*/
protected function render() {
$settings = $this->get_settings_for_display();
if ( empty( $settings['url'] ) ) {
return;
}
$html = wp_oembed_get( $settings['url'] );
?>
<div class="oembed-elementor-widget">
<?php echo ( $html ) ? $html : $settings['url']; ?>
</div>
<?php
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
# The Result
A new "oEmbed" widget:
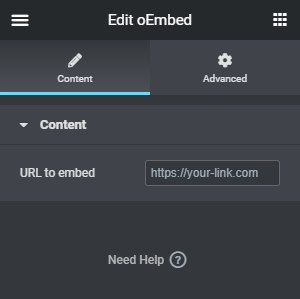