# Advanced Example
Elementor Core BasicFor a more advanced use-case, we'll replace static links with core WordPress functions, linking to the WordPress dashboard settings panels.
# Folder Structure
The addon will have two files. One file for the finder category and a main file to register the class.
elementor-finder-wordpress-settings/
|
├─ finder/
| └─ wordpress-settings.php
|
└─ elementor-finder-wordpress-settings.php
1
2
3
4
5
6
2
3
4
5
6
# Plugin Files
elementor-finder-wordpress-settings.php
<?php
/**
* Plugin Name: Elementor Finder WordPress Settings
* Description: Custom WordPress settings links in Elementor Finder.
* Plugin URI: https://elementor.com/
* Version: 1.0.0
* Author: Elementor Developer
* Author URI: https://developers.elementor.com/
* Text Domain: elementor-finder-wordpress-settings
*
* Requires Plugins: elementor
* Elementor tested up to: 3.25.0
* Elementor Pro tested up to: 3.25.0
*/
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
/**
* Add custom Finder categories.
*
* Include finder file and register the class.
*
* @since 1.0.0
* @param \Elementor\Core\Common\Modules\Finder\Categories_Manager $finder_categories_manager.
* @return void
*/
function elementor_finder_wordpress_settings( $finder_categories_manager ) {
require_once( __DIR__ . '/finder/wordpress-settings.php' );
$finder_categories_manager->register( new Elementor_Finder_WordPress_Settings() );
};
add_action( 'elementor/finder/register', 'elementor_finder_wordpress_settings' );
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
finder/social-media.php
<?php
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
/**
* Elementor Finder - WordPress Settings
*
* Provides searchable items related to WordPress settings.
*/
class Elementor_Finder_WordPress_Settings extends \Elementor\Core\Common\Modules\Finder\Base_Category {
/**
* Get finder category id.
*
* @since 1.0.0
* @access public
* @return string Finder category id.
*/
public function get_id(): string {
return 'wordpress-settings';
}
/**
* Get finder category title.
*
* @since 1.0.0
* @access public
* @return string Finder category title.
*/
public function get_title(): string {
return esc_html__( 'WordPress Settings', 'elementor-finder-wordpress-settings' );
}
/**
* Get finder category items.
*
* @since 1.0.0
* @access public
* @param array $options
* @return array An array of category items.
*/
public function get_category_items( array $options = [] ): array {
return [
'general' => [
'title' => esc_html__( 'General', 'elementor-finder-wordpress-settings' ),
'icon' => 'wordpress',
'url' => admin_url( 'options-general.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'general', 'settings' ],
],
'writing' => [
'title' => esc_html__( 'Writing', 'elementor-finder-wordpress-settings' ),
'icon' => 'edit',
'url' => admin_url( 'options-writing.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'writing', 'settings' ],
],
'reading' => [
'title' => esc_html__( 'Reading', 'elementor-finder-wordpress-settings' ),
'icon' => 'post-content',
'url' => admin_url( 'options-reading.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'reading', 'settings' ],
],
'discussion' => [
'title' => esc_html__( 'Discussion', 'elementor-finder-wordpress-settings' ),
'icon' => 'comments',
'url' => admin_url( 'options-discussion.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'discussion', 'settings' ],
],
'media' => [
'title' => esc_html__( 'Media', 'elementor-finder-wordpress-settings' ),
'icon' => 'image',
'url' => admin_url( 'options-media.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'media', 'settings' ],
],
'permalink' => [
'title' => esc_html__( 'Permalink', 'elementor-finder-wordpress-settings' ),
'icon' => 'editor-link',
'url' => admin_url( 'options-permalink.php' ),
'keywords' => [ 'wordpress', 'dashboard', 'permalink', 'settings' ],
],
];
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
# The Result
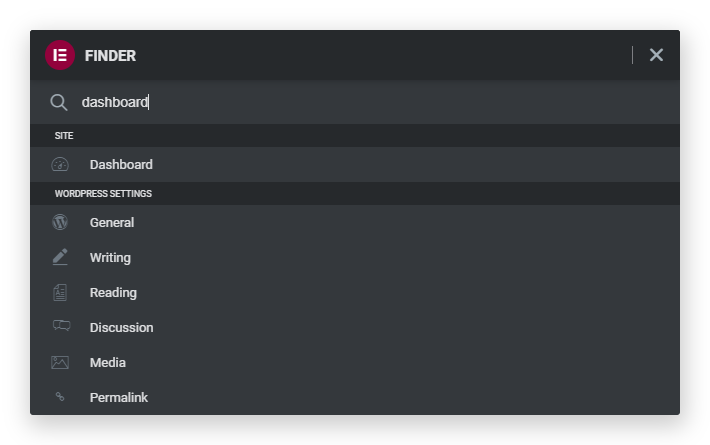