# Simple Example
Elementor Core BasicIn this example, we'll build a full Elementor addon to modify a context menu.
# Folder Structure
The addon will have two files. The main file will register and enqueue a JS file in the editor, and the JS will modify the context menu.
elementor-context-menus/
|
├─ assets/js/
| └─ context-menus.js
|
└─ elementor-context-menus.php
1
2
3
4
5
6
2
3
4
5
6
# Plugin Files
elementor-context-menus.php
<?php
/**
* Plugin Name: Elementor Context Menus
* Description: Custom context menus for Elementor page builder.
* Plugin URI: https://elementor.com/
* Version: 1.0.0
* Author: Elementor Developer
* Author URI: https://developers.elementor.com/
* Text Domain: elementor-context-menus
*
* Requires Plugins: elementor
* Elementor tested up to: 3.21.0
* Elementor Pro tested up to: 3.21.0
*/
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
function elementor_context_menus_scripts() {
wp_enqueue_script(
'elementor-context-menus',
plugins_url( 'assets/js/context-menus.js', __FILE__ ),
[],
'1.0.0',
false
);
}
add_action( 'elementor/editor/after_enqueue_scripts', 'elementor_context_menus_scripts' );
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
assets/js/context-menus.js
window.addEventListener( 'elementor/init', () => {
elementor.hooks.addFilter( 'elements/context-menu/groups', ( customGroups, elementType ) => {
const newGroup = {
name: 'elementor-links-group',
actions: [
{
name: 'elementor-link',
icon: 'eicon-link',
title: 'Elementor.com',
isEnabled: () => true,
callback: () => window.open( 'https://elementor.com/', '_blank' ).focus(),
},
{
name: 'elementor-developers-link',
icon: 'eicon-link',
title: 'Developers Docs',
isEnabled: () => true,
callback: () => window.open( 'https://developers.elementor.com/', '_blank' ).focus(),
}
],
}
if ( 'widget' === elementType ) {
customGroups.push( newGroup );
}
return customGroups;
} );
} );
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
# The Result
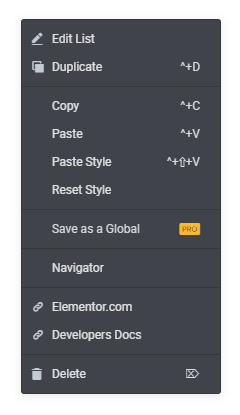