# Custom Query Filter
Elementor Pro AdvancedBoth posts widgets and portfolio widgets come with a robust query control that lets you select specific posts to show in the widget. But sometimes, you need more control over the query. For those situations, there is the custom query filter, which exposes the WP_Query (opens new window) object and allows you to customize the query in any way you want.
# Hook Details
- Hook Type: Action Hook
- Hook Name:
elementor/query/{$query_id}
- Affects: Query
# Hook Arguments
Argument | Type | Description |
---|---|---|
query | \WP_Query | The WordPress query instance. |
widget | \Elementor\Widget_Base | The widget instance. |
# Setting Up a Custom Filter
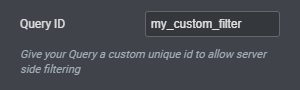
In a posts or portfolio widget, give your query an ID, making sure it is unique, unless you want the filter to run on multiple posts or portfolio widgets.
In the examples below, the query ID is set to my_custom_filter
, so when Elementor renders the widget, it will create a custom filter based on the query ID: elementor/query/my_custom_filter
.
# Using the Custom Filter
After you have set up the custom query filter, you can use it to modify the query in the same way that the WordPress native pre_get_posts (opens new window) hook lets you modify a query. Using the custom query filter is just like any other WordPress native action hook:
/**
* Update the posts widget or portfolio widget query.
*
* @since 1.0.0
* @param \WP_Query $query The WordPress query instance.
*/
function custom_query_callback( $query ) {
// Modify the posts query here
}
add_action( 'elementor/query/{$query_id}', 'custom_query_callback' );
2
3
4
5
6
7
8
9
10
# Examples
# Multiple Post Types in a Posts Widget
Use the following code snippet to show multiple post types in a posts widget:
/**
* Update the query to use specific post types.
*
* @since 1.0.0
* @param \WP_Query $query The WordPress query instance.
*/
function my_query_by_post_types( $query ) {
$query->set( 'post_type', [ 'custom-post-type1', 'custom-post-type2' ] );
}
add_action( 'elementor/query/{$query_id}', 'my_query_by_post_types' );
2
3
4
5
6
7
8
9
10
# Filter Posts by the Posts' Metadata in a Portfolio Widget
Use the code below to show posts with a metadata key filter in a portfolio widget:
/**
* Update the query by specific post meta.
*
* @since 1.0.0
* @param \WP_Query $query The WordPress query instance.
*/
function my_query_by_post_meta( $query ) {
// Get current meta Query
$meta_query = $query->get( 'meta_query' );
// If there is no meta query when this filter runs, it should be initialized as an empty array.
if ( ! $meta_query ) {
$meta_query = [];
}
// Append our meta query
$meta_query[] = [
'key' => 'project_type',
'value' => [ 'design', 'development' ],
'compare' => 'in',
];
$query->set( 'meta_query', $meta_query );
}
add_action( 'elementor/query/{$query_id}', 'my_query_by_post_meta' );
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
# Most Popular Post by Comment Count in a Posts Widget
Use the following to show posts ordered by comment count in a posts widget:
/**
* Order the posts in the query by comment count.
*
* @since 1.0.0
* @param \WP_Query $query The WordPress query instance.
*/
function my_query_by_different_order( $query ) {
$query->set( 'orderby', 'comment_count' );
}
add_action( 'elementor/query/{$query_id}', 'my_query_by_different_order' );
2
3
4
5
6
7
8
9
10
# Show Posts with Multiple Statuses in a Posts Widget
Use the following to show posts by specific post statuses:
NOTE: Using this snippet may result in displaying private data. Please use with caution.
/**
* Update the query to use specific post statuses.
*
* @since 1.0.0
* @param \WP_Query $query The WordPress query instance.
*/
function my_query_by_post_status( $query ) {
$query->set( 'post_status', [ 'future', 'draft'] );
}
add_action( 'elementor/query/{$query_id}', 'my_query_by_post_status' );
2
3
4
5
6
7
8
9
10
# Notes
You may need to refresh the editor to see the filter's effect.